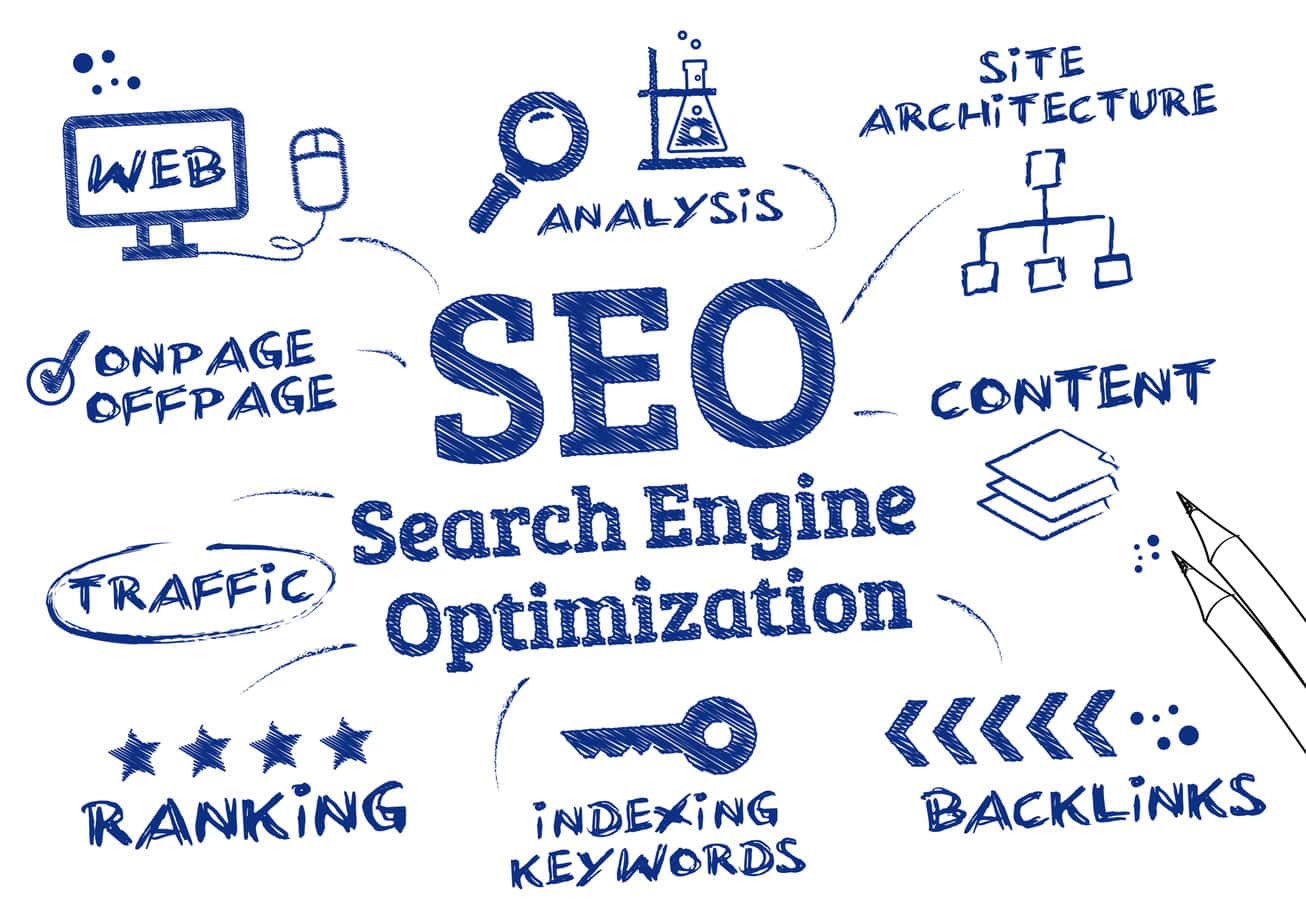
SEO optimization for nextjs
Gerardo Nastri / October 3, 2024
SEO, and specifically the on-page SEO we'll be looking at today, is about optimizing the content within your site, such as proper keyword usage, title structure, meta tags, site speed, and accessibility.
Leverage Server-Side Rendering (SSR)
Server-Side Rendering (SSR) in Next.js is a technique where web pages are generated and rendered directly on the server, rather than on the client side. This means that when a user requests a page, the server dynamically generates the HTML content and sends it ready-made to the browser, rather than just sending JavaScript code that the browser must execute to generate the content.
export default function Home() {
const res = await fetch(`${URL}/resources`);
const data = await res.json();
return <h1>{data[0].title}</h1>
}
Optimize Meta Tags and Titles
Meta tags and titles play a vital role in SEO, influencing how your pages are displayed in search results. Next.js's Head component allows you to dynamically set these tags per page, improving relevancy and click-through rates.
import Head from 'next/head';
function HomePage() {
return (
<>
<Head>
<title>Your Page Title</title>
<meta name="description" content="A brief description of your page" />
</Head>
{/* Page content */}
</>
);
}
Image optimization
Use Next.js's image-optimized component to reduce loading times without sacrificing image quality, which has a positive impact on SEO. Remember to use alt!!
import Image from 'next/image';
function HomePage() {
return (
<>
<Image src="your-image.webp" alt="logo image" width={50} height={50} />
</>
);
}
Correct use of HTML tags
Properly organize your heading tags (H1, H2, H3, etc.) to improve page structure and make it easier for search engines to crawl.
Meta tag e metadata optimization
Add appropriate meta tags, including those for the title and description, as well as generate additional metadata to improve display in search results and on social media.
import type { Metadata } from "next";
import "./globals.css";
export const metadata: Metadata = {
title: "Blog SEO",
description: "Generated by create next app",
other: {
"theme-colo": "#od1117",
"color-scheme": "dark-only",
},
};
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<html lang="en">
<body className="min-h-screen bg-black-100 font-poppins">{children}</body>
</html>
);
}
Technologies used
These are the technologies I used for this website.
- Next.js: A React framework for building JavaScript applications.
- Sanity.io: A platform for structured content that comes with an open-source editing environment called Sanity Studio.
- Tailwind CSS: A utility-first CSS framework for rapidly building custom user interfaces.
- TypeScript: A statically typed superset of JavaScript that adds optional types.
- React: A JavaScript library for building user interfaces.
- Node.js: A JavaScript runtime built on Chrome's V8 JavaScript engine.
- CSS: A stylesheet language used for describing the look and formatting of a document written in HTML.
- JSON: A lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate.
- @sanity/image-url: A library for generating image URLs with Sanity.io.
- next-sanity: A library that provides utilities for working with Sanity.io in Next.js applications.
- clsx: A tiny utility for constructing className strings conditionally.
- tailwind-merge: A utility for merging Tailwind CSS classes.
- query-string: A library for parsing and stringifying URL query strings.
How to use Nexjs
To use NextJs, you need to create a new NextJs project by running the following command:
npx create-next-app my-app
This will create a new NextJs project in a directory called my-app
. You can
then navigate to the project directory and start the development server by
running the following command:
cd my-app
npm run dev
This will start the development server and open your NextJs application in your browser. You can then start building your application by creating new pages, components, and API routes.
Conclusion
NextJs is a powerful React framework that makes it easy to build server-rendered React applications. This post has introduced you to the basics of SEO on-page and explained how you want to use it in your projects.