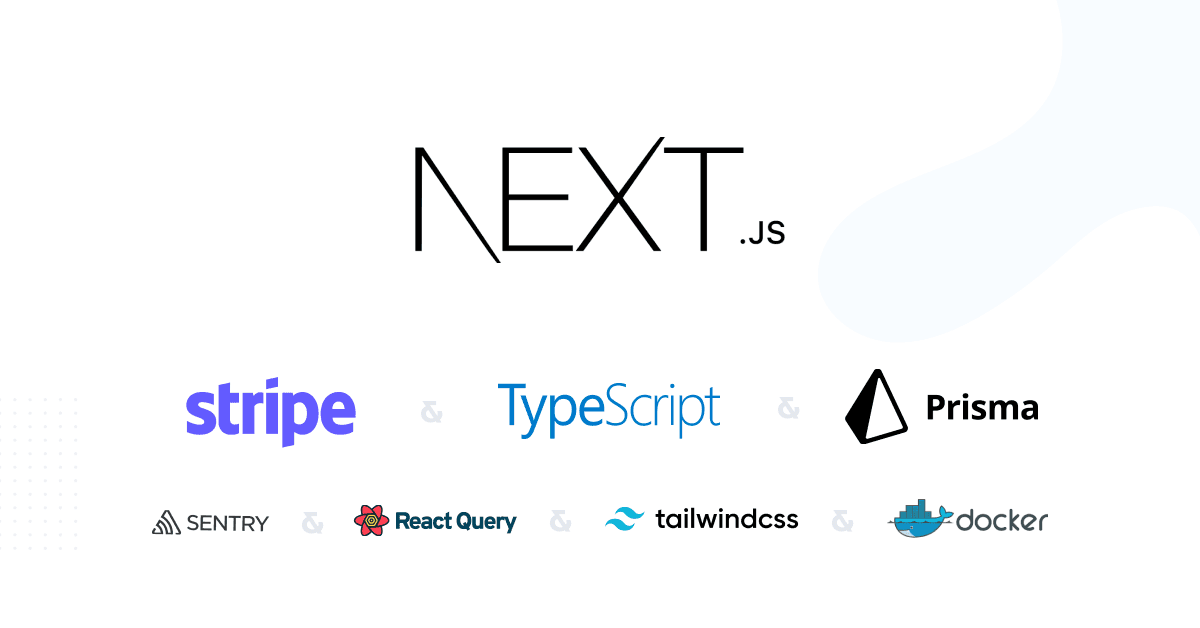
Next js Full Stack for Best Results
Gerardo Nastri / October 8, 2024
The Ultimate Tech Stack for Building High-Performance Applications with Next.js
As a full-stack developer, I’ve worked with various technologies and frameworks, but Next.js remains one of my favorites due to its flexibility, performance, and ease of use. In this article, I’ll walk you through my preferred tech stack when building applications with Next.js, focusing on tools and libraries that help enhance development speed, scalability, and maintainability.
1. Next.js: The Foundation of Modern Web Development
Next.js is a React framework that offers server-side rendering, static site generation, and API routes, all out of the box. This framework is perfect for building SEO-friendly applications with superior performance.
2. Tailwind CSS: Utility-First Styling
For managing styles, I use Tailwind CSS, a utility-first CSS framework that allows me to create fully responsive and highly customized UI components. Tailwind's approach lets me focus on writing minimal custom CSS, making the styling process quick and scalable.
For example, with Tailwind, you can build a responsive card component in just a few lines:
<div className="max-w-sm rounded overflow-hidden shadow-lg">
<img className="w-full" src="/img/card-top.jpg" alt="Card image cap" />
<div className="px-6 py-4">
<div className="font-bold text-xl mb-2">Card Title</div>
<p className="text-gray-700 text-base">Card content goes here.</p>
</div>
</div>
3. TypeScript: Type Safety and Scalability
While JavaScript is powerful, TypeScript takes it to another level. TypeScript ensures that the code I write is type-safe, making it easier to maintain large-scale projects. By catching potential errors at compile-time rather than runtime, it boosts development productivity and code reliability.
4. Firebase: Real-Time Database and Authentication
For real-time data management, Firebase is my go-to solution. It provides real-time database capabilities and user authentication, allowing me to easily handle user sessions and data updates across multiple clients in real-time.
One example where Firebase shines is in building a real-time chat feature. Using Firebase's real-time database, I can easily synchronize chat messages across different devices without complex backend logic.
5. Supabase: The Open-Source Backend
While Firebase works great for real-time use cases, I often pair Supabase with Next.js for traditional CRUD operations. Supabase offers an open-source alternative to Firebase, built on top of PostgreSQL. It provides easy database management and a rich set of features such as authentication, storage, and real-time subscriptions.
For example, integrating Supabase with Next.js API routes allows for straightforward data fetching and manipulation:
import { createClient } from '@supabase/supabase-js';
const supabase = createClient('your-supabase-url', 'your-supabase-key');
export async function getServerSideProps() {
const { data, error } = await supabase.from('your-table').select('*');
return { props: { data } };
}
6. Framer Motion: Beautiful Animations
For animations and transitions, Framer Motion is my go-to library. It integrates smoothly with React and Next.js, allowing for elegant and intuitive animations that enhance the user experience.
Here's a simple example of animating a button hover effect using Framer Motion:
<motion.button
whileHover={{ scale: 1.1 }}
whileTap={{ scale: 0.9 }}
className="bg-blue-500 text-white px-4 py-2 rounded"
>
Click Me
</motion.button>
7. GraphQL and Apollo: Efficient Data Management
When building applications that require flexible and efficient data fetching, I opt for GraphQL along with the Apollo Client. GraphQL allows clients to request exactly the data they need, reducing over-fetching and improving performance, especially in large applications.
For instance, in a Next.js app, Apollo Client can be set up to query data from a GraphQL API easily:
import { ApolloClient, InMemoryCache, gql } from '@apollo/client';
const client = new ApolloClient({
uri: 'https://your-graphql-endpoint.com',
cache: new InMemoryCache(),
});
export async function getServerSideProps() {
const { data } = await client.query({
query: gql`
query GetItems {
items {
id
name
price
}
}
`,
});
return { props: { data } };
}
8. NextAuth.js: Flexible Authentication
Managing authentication can be challenging, but NextAuth.js makes it simple by providing an easy-to-configure solution that integrates seamlessly with Next.js. Whether you need OAuth-based login (like Google or GitHub) or a custom authentication system, NextAuth.js handles it effortlessly.
For example, integrating Google authentication would look like this:
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
],
});
9. Stripe: Secure Payments
For e-commerce applications, Stripe is the de facto choice for handling online payments. Its seamless API and developer-friendly documentation make it easy to integrate secure and scalable payment systems in Next.js applications.
A simple checkout flow using Stripe can be implemented as follows:
import { loadStripe } from '@stripe/stripe-js';
const stripePromise = loadStripe('your-publishable-key');
const handleCheckout = async () => {
const stripe = await stripePromise;
await stripe.redirectToCheckout({ sessionId: 'your-session-id' });
};
10. Vercel: Effortless Deployment
Last but not least, I use Vercel for deploying Next.js applications. Since Vercel is the team behind Next.js, it offers seamless integration, automatic scaling, and optimizations that make deploying serverless applications a breeze.
Conclusion
This tech stack has proven to be powerful and flexible, allowing me to build robust, scalable, and high-performance applications with Next.js at the core. Whether it's handling user authentication, managing real-time data, or integrating sleek animations, these tools ensure my projects are modern, fast, and maintainable. By combining these technologies, I can build applications that not only perform well but also provide an exceptional user experience.