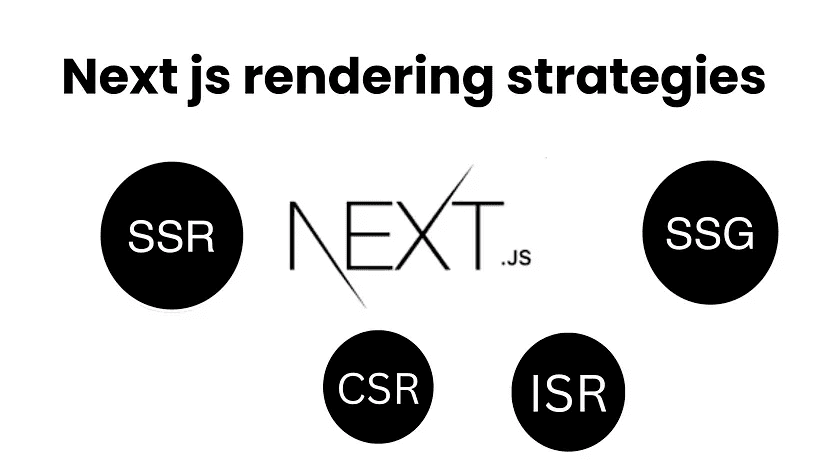
Next js 15 rendering methods
Gerardo Nastri / November 3, 2024
Rendering Methods in Next.js 15: MDX, PPR, SSR, SSG, Client Rendering, and ISR
Next.js 15 introduces improvements and modern practices, utilizing the app
directory and advanced rendering options. Below, we explore examples for PPR, SSR, SSG, client-side rendering, and ISR.
1. SSR (Server-Side Rendering)
Description: Server-Side Rendering (SSR) generates pages on the server for each request. This means that every time a user visits a page, the server renders the page in real-time and sends the fully rendered HTML content to the client.
Advantages:
- Great for SEO as search engines can easily index pre-rendered pages.
- Useful for dynamic content that changes frequently.
Disadvantages:
- Performance can be slower compared to other solutions since each request requires complete server-side rendering.
// app/ssr-example/page.tsx
async function SSRExamplePage() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return (
<div>
<h1>Server-Side Rendered Page</h1>
<p>{data.message}</p>
</div>
);
}
2. SSG (Static Site Generation)
Description: Static Site Generation (SSG) pre-renders pages at build time, generating static HTML files. These pages are then served directly to clients, resulting in very fast load times.
Advantages:
- High speed and performance since static pages are served as simple HTML files.
- Scalability: Ideal for sites with content that doesn't change frequently (e.g., blogs, documentation).
Disadvantages:
- Not suitable for frequently changing content without using a strategy like ISR.
// app/ssg-example/page.tsx
async function SSGExamplePage() {
const response = await fetch('https://api.example.com/data', {
cache: 'force-cache',
});
const data = await response.json();
return (
<div>
<h1>Static Site Generated Page</h1>
<p>{data.message}</p>
</div>
);
}
export default SSGExamplePage;
3. CSR (Client-Side Rendering)
Description: Client-Side Rendering (CSR) involves downloading a basic HTML page and loading JavaScript to dynamically render content on the client side. React handles the rendering logic after the initial page load.
Advantages:
- Smooth user experience for highly interactive applications.
- Data fetching can occur in the background after the initial page load.
Disadvantages:
- Limited SEO as search engines might struggle to index content loaded via JavaScript.
- Slower initial load compared to SSR and SSG, as more work is done on the client.
'use client';
import { useEffect, useState } from 'react';
const ClientRenderingExample = () => {
const [data, setData] = useState(null);
useEffect(() => {
async function fetchData() {
const response = await fetch('/api/data');
const result = await response.json();
setData(result);
}
fetchData();
}, []);
return (
<div>
<h1>Client-Side Rendered Page</h1>
{data ? <p>{data.message}</p> : <p>Loading...</p>}
</div>
);
};
export default ClientRenderingExample;
4. ISR (Incremental Static Regeneration)
Description: Incremental Static Regeneration (ISR) is a hybrid of SSG and CSR. It allows for updating static pages after the initial build at specified intervals without regenerating the entire site.
Advantages:
- Dynamic updates of static content without having to rebuild the entire site.
- Performance close to SSG with the benefit of periodic updates.
Disadvantages:
- Can be complex to set up properly for content that requires controlled regeneration.
// app/isr-example/page.tsx
async function ISRExamplePage() {
const response = await fetch('https://api.example.com/data', {
next: { revalidate: 60 }, // Revalidate every 60 seconds
});
const data = await response.json();
return (
<div>
<h1>Incremental Static Regeneration Page</h1>
<p>{data.message}</p>
</div>
);
}
export default ISRExamplePage;
When to Use Each Method?
- SSR: Best for pages that need fresh, up-to-date content on each request, such as real-time data pages.
- SSG: Ideal for stable content, such as blogs or documentation, where content does not change frequently.
- CSR: For highly interactive applications that need full control of client-side data rendering.
- ISR: Perfect for static content that benefits from occasional updates without the need for a complete site rebuild.